Last Update:
C++ at the end of 2012
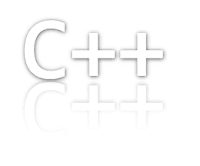
Table of Contents
This year (2012) and the previous one were good time for C++ language. We have the new standard: C++0x became C++11. What is more important is that the language will definitely not be forgotten and new ideas and plans are coming! Another key thing is that Cpp is used in a lot of new spaces - for instance in WinRT, C++ AMP, QT5 with C++11 support and more. Hopefully the language will be accepted and I will not loose my job any time soon :)
Other Reports:
2020 2019, 2018, 2017, 2016, 2015, 2014, 2013, 2012.
Cool stuff
I will not be creative and I simply paste this quote:
“Surprisingly, C++11 feels like a new language” - C++11 FAQ from Stroustrup.
So far I did not code using C++11 much. I have read a lot of articles and watched several presentation on that case. I need to learn quite a few new techniques and concepts to fully use the new standard. Fortunately this seems to be quite easy and nice journey. Hope I will be soon able to update my project to use new Cpp. All in all I can agree with the quote above.
Lambdas, initializer lists, uniform initialization, for each, member initialization, nullptr, static assert, raw string literals, auto, etc, etc - those keywords make only syntactic sugar. What is more significant is that programming style should be fresher, newer and easier. Lets us see some simple code:
std::string createUniqeName()
{
std::string strName;
// make unique name...
return strName;
}
// somewhere in the code
std::string name = createUniqeName();
Idea of this code was grabbed from one of Herb Sutter’s lectures. This short piece of code shows quite silly thing. But it C++98 you would probably wonder whether to use pointer or maybe a reference, or maybe return by argument… using value type would have been too expensive for sure. But now with “move” semantics this piece of code should be as fast as when using pointers! Another advantage is that you simply do not have to bother, just use return by value. Under the hood string will not be copied but actually moved - using a move constructor. This, I think, is quite a fresh idea that makes Cpp more user-friendly.
What about some more examples?
// isn't that much simplier and so obvious?
vector <int> vec = { 0, 1, 2, 3, 4, 5 };
// much shorter than using separate functor object:
sort(vec.begin(), vec.end(), \[\](const int& a, const int& b) -> bool
{
return a > b;
});
// add any nice C++11 code here :)
We could add some more source code here. But the point is that the language and its syntax ‘caught’ modern standard and should be a bit better to use.
Ideas
Let us take a look on some motivations that are base of of working with C++.
- close to hardware - you can do almost everything you want, but it comes with some price - you must know what you are doing.
- performance and control - you have control over the hardware and your data. If you want you can use garbage collector but this is your choice.
- memory - decide where are your objects stored. Much simpler with new smart pointers and when learnt properly you can use them as working with some managed language.
- trust the programmer - C++ is hard to learn, but when you know it you have a great power over your code. On the other hand it is quite simple to ruin the code and make the software more buggy.
Performance
Is performance still important? We might say that computers today are so fast that I do not have to worry about every code instruction, I can use interpreted language and be more productive instead. Moreover not so many programmers work in CERN, on scientific computation, hardcore graphics engines, games engines, etc. Of course… but what about phones, tablets and all those mobile devices? What about ultrabooks? All of those devices need power efficient software. So maybe it would be nice to built them in C++? We can see, for instance, some encouragement from M$ that enabled C++ native development on WinRT
Not to mention that most of server code - in Google, Facebook, etc is also written in C/C++. All in all we see that there is still much space left for C++ code and we cannot all go and use Python and JavaScript.
Roadmap
Fortunately C++11 is not the final version. After watching “The Future of C++” I see that C++ committee is and will be working pretty hard to give us updates and new standards. We needed to wait 13 years - between C++98 and C++11. But now a new major version of the standard is planned for 2017, before that several minor releases are planned as well.
Another thing is that the “Standard C++ Foundation” was created. Its official site is isocpp.org. Now we can get all the knowledge from single place. Before that news, standards and tutorials were scattered across the net.
Problems
The new language is pretty cool, but C++ in general still has some problems:
- legacy code - Cpp is not that fancy and cool as new interpreted languages like Python for instance. Most of us can link Cpp with legacy code only and at start we are discouraged and do not have motivation to learn. I agree that legacy code is horrible, but somehow we need to live with that and maybe refactor this code into new standard. Maybe it will not take hundreds of years :)
- adaptation - new standard is cool, but even now we do not have compilers that fully support it! After compilers programmers need to adapt. This will take years. You can create your small projects with new standard, but for large companies with huge projects this will definitely take some time.
- learning - as said before - Cpp is quite hard to learn. New standard improves situation a bit, but still when you want to have a C++ job you have to know the ‘old’ standard. This is not easy and people will probably chose simpler languages.
- low level - it is great that we can create almost everything in Cpp, but is this really fastest way of doing that? Sometimes you wonder too much about the internal stuff before writing the code, this takes some time and can create bugs.
- tools - syntax and the structure of the language is very complicated and there is not so many good tools that improve coding. Although there are lot of powerful refactoring tools for C# and Java there is only a little for C++. Even IntelliSense and other code auto-completion tools even now are quite buggy and work worse than those we can find in .NET for instance. Overall tools makes learning and living with Cpp much harder that it should be.
- standard library - although there is standard library for C++ it is not that big as for Java and .NET. We need to use some third party software and that sometimes increases overall production time. With the new C++11 the standard library gets bigger, so maybe this will improve that situation.
For a defence of C++ we need to consider that C++ gives us a lot of power, a lot of native power. But that comes with a cost. It cannot be used for all problems and maybe for your particular problem C# or Python would be much better.
Conclusion
Cpp was refreshed and that is a very good sign. I expect to see real projects that fully utilize new features and can prove that new standard is really great and allows us to make better software. Unfortunately still C++ is often treated as a tool only for the legacy code. I hope that the next year will be even better for the language :)
To see: isocpp.org - main site for Cpp
Links
- Herb Sutter - (Not your father’s) C++ - short (45 min) presentation about current status of C++ and comparison with “managed” languages.
- The Future of C++ - another presentation from Herb Sutter
- C++11 FAQ - FAQ from Bjarne Stroustrup
- simpleprogrammer.com/2012/12/01/why-c-is-not-back/ - interesting post and a discussion about problems with C++
- C++ 11 vs C++03 by Alex Sinyakov (pdf) - lots of slides with comparison between new and the old version of standard. This is pdf stored on dropbox, so this link can be inactive in some time.
PS: fortunately I got in time and posted this entry before end of the year :)
I've prepared a valuable bonus for you!
Learn all major features of recent C++ Standards on my Reference Cards!
Check it out here: