Last Update:
C++ (Core) Coding Guidelines
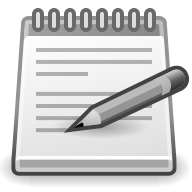
Table of Contents
Since 2011, when C++11 arrived, we all should be changing our coding style into modern C++ and at the same time keep good old tips. There are also general rules for programming and bug-free coding. Here’s a list of guidelines and other resources that might help you.
Core C++ Guidelines
Main site: C++ Core Guidelines
From the abstract:
This document is a set of guidelines for using C++ well. The aim of
this document is to help people to use modern C++ effectively. By
“modern C++” we mean C++11 and C++14 (and soon C++17). In other words,
what would you like your code to look like in 5 years’ time, given
that you can start now? In 10 years’ time?
Elements:
- In: Introduction
- P: Philosophy
- I: Interfaces
- F: Functions
- C: Classes and class hierarchies
- Enum: Enumerations
- R: Resource management
- ES: Expressions and statements
- E: Error handling
- Con: Constants and immutability
- T: Templates and generic programming
- CP: Concurrency
- STL: The Standard library
- SF: Source files
- CPL: C-style programming
- PRO: Profiles
- GSL: Guideline support library
- FAQ: Answers to frequently asked questions
- NL: Naming and layout
- PER: Performance
- N: Non-Rules and myths
- RF: References
- Appendix A: Libraries
- Appendix B: Modernizing code
- Appendix C: Discussion
The guideline is moderated by Bjarne Stroustrup and Herb Sutter. Here’s Bjarne Stroustrup’s C++ Style and Technique FAQ and Sutter’s GotW section.
Additionally here’s the post from Kate Gregory about coding guidelines: C++ Core Guidelines and Checking Tool And also, she started doing more posts about core guidelines, for example: Using the not_null Template for Pointers That Must Never Be Null
Learn from bugs
Some time ago a company that produces PVS-Studio for C/C++/C# (viva64.com/) posted a very long list of potential bugs and tips that you can use to improve your C++ code. It’s also in the form of a book:
The book covers 42 topics. In spite of the simple titles of the
chapters, the bugs found are really various and non-standard. In
addition to that, the text provides a lot of links to interesting
materials that give more details on topics. To make more use of this
book, please don’t hurry and go to the links provided.
Content:
- Don’t do the compiler’s job
- Larger than 0 does not mean 1
- Copy once, check twice
- Beware of the ?: operator and enclose it in parentheses
- Use available tools to analyze your code
- Check all the fragments where a pointer is explicitly cast to integer types
- Do not call the alloca() function inside loops
- Remember that an exception in the destructor is dangerous.
- Use the ‘\0’ literal for the terminal null character
- Avoid using multiple small #ifdef blocks
- Don’t try to squeeze as many operations as possible in one line
- When using Copy-Paste, be especially careful with the last lines
- Table-style formatting
- A good compiler and coding style aren’t always enough
- Start using enum class in your code, if possible
- “Look what I can do!” - Unacceptable in programming
- Use dedicated functions to clear private data
- The knowledge you have, working with one language isn’t always applicable to another language
- How to properly call one constructor from another
- The End-of- file (EOF) check may not be enough
- Check that the end-of- file character is reached correctly (EOF)
- Do not use #pragma warning(default:X)
- Evaluate the string literal length automatically
- Override and final identifiers should become your new friends.
- Do not compare ’this’ to nullptr anymore
- Insidious VARIANT_BOOL
- Guileful BSTR strings
- Avoid using a macro if you can use a simple function
- Use a prefix increment operator (++i) in iterators instead of a postfix (i++) operator
- Visual C++ and wprintf() function
- In C and C++ arrays are not passed by value
- Dangerous printf
- Never dereference null pointers
- Undefined behavior is closer than you think
- Adding a new constant to enum don’t forget to correct switch operators
- If something strange is happening to your PC, check its memory.
- Beware of the ‘continue’ operator inside do {…} while (…)
- Use nullptr instead of NULL from now on
- Why incorrect code works
- Start using static code analysis
- Avoid adding a new library to the project.
- Don’t use function names with “empty”
Here’s the post: http://www.viva64.com/en/b/0391/ that gets regularly updated. I highly encourage you to read about those problems from time to time… maybe something similar could be improved in your apps?
Google Coding Standard
Google C++ Coding Standard is another popular resource that is public and easy to find
Just go here: https://google.github.io/styleguide/cppguide.html
Top level index:
- Header Files
- Scoping
- Classes
- Functions
- Google-Specific Magic
- Other C++ Features
- Naming
- Comments
- Formatting
- Exceptions to the Rules
- Existing Non-conformant CodeWindows Code
Since Google is a software giant, we should obey their rules, right? Not that easy! There is a huge discussion going on if the guide is good or not. Read especially this detailed post: Why Google Style Guide for C++ is a deal-breaker. And here is a reddit thread for the article.
What are the main controversies over the guide: banning exceptions, public inheritance, passing reference parameters, probably not using advanced template techniques.
To see reasoning behind the guideline, you can watch this video:
CppCon 2014: Titus Winters “The Philosophy of Google’s C++ Code”
Other guidelines
Bloomberg - BDE
https://github.com/bloomberg/bde/wiki/Introduction-to-BDE-Coding-Standards
OpenOffice
http://www.openoffice.org/tools/coding.html
LLVM
http://llvm.org/docs/CodingStandards.html
Mozilla
https://developer.mozilla.org/en-US/docs/Mozilla/Developer_guide/Coding_Style
Chrominium
https://www.chromium.org/developers/coding-style
Mostly it uses Google Style Guide, but here are also some specific sections: C++11 use in Chromium or C++ Dos and Don’ts
High Integrity C++ Coding Standard Version
http://www.codingstandard.com/
WebKit
https://webkit.org/code-style-guidelines/
QT
https://wiki.qt.io/Coding_Conventions
ROS (Robot Operating System)
http://wiki.ros.org/CppStyleGuide
They have also invested in auto format tool: roscpp Code Format
SEI CERT C++ Coding Standard
Little Bonus:
- Linux Kernel Guideline C language - @kernel.org
- NASA JPL Guideline - C language, PDF - PDF guideline and the reddit discussion.
Books
- Effective C++ series from Scott Meyers
- C++ Coding Standards: 101 Rules, Guidelines, and Best Practices, from 2004, but still contains some useful tips.
Blog posts
- Richard Rodger: Why I Have Given Up on Coding Standards
- CodeAhoy: Effective Coding Standards
- Paul M. Jones: Why Coding Standards Matter
Summary
In this post, I brought you a list of c++ guidelines that might add value to your internal guidelines. Please have a look especially at C++ Core Guidelines since it’s created by the community and moderated by Bjarne Stroustrup and Herb Sutter.
What guideline I am missing here? Let me know if you have a useful link to that.
- What coding guideline do you use? Company internal? Or some open guideline?
- Do you obey the rules from your guideline?
- Do you use auto format tools?
I've prepared a valuable bonus for you!
Learn all major features of recent C++ Standards on my Reference Cards!
Check it out here: