Last Update:
Errata and a Nice C++ Factory Implementation
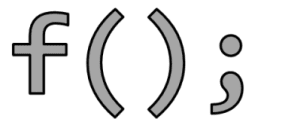
I’ve finally got my copy of “Effective Modern C++”! The book looks great, good paper, nice font, colors… and of course the content :)
While skimming through it for the first (or second) time I’ve found a nice idea for a factory method. I wanted to test it.
The idea
In the Item 18 there was described how to use std::unique_ptr
and why
it’s far better than raw pointers or (deprecated) auto_ptr
.
As an example there was a factory presented:
template<typename... Ts>
std::unique_ptr<Investment>
makeInvestment(Ts&&... params);
Looked nice! I thought, that I would be able to return unique pointers to proper derived classes. The main advantage: variable count of parameters for construction. So one class might have one parameter, another could have three, etc, etc…
I’ve quickly create some code:
template <typename... Ts>
static std::unique_ptr<IRenderer>
create(const char *name, Ts&&... params)
{
std::string n{name};
if (n == "gl")
return std::unique_ptr<IRenderer>(
new GLRenderer(std::forward<Ts>(params)...));
else if (n == "dx")
return std::unique_ptr<IRenderer>(
new DXRenderer(std::forward<Ts>(params)...));
return nullptr;
}
But then when I tried to use it:
auto glRend = RendererFactory::create("gl", 100);
auto dxRend = RendererFactory::create("dx", 200, DX_11_VERSION);
It did not compile…
gcc 4.9.1:
factory.cpp:28:7: note: constexpr GLRenderer::GLRenderer(GLRenderer&&)
factory.cpp:28:7: note: candidate expects 1 argument, 2 provided
There is no way to compile it. All constructors would have to have the same number of parameters (with the same types).
Errata
Then I’ve found errata for the book: Errata List for Effective Modern C++
The
makeInvestment
interface is unrealistic, because it implies that all derived object types can be created from the same types of arguments. This is especially apparent in the sample implementation code, where are arguments are perfect-forwarded to all derived class constructors.
hmm… that would be all.
Such a nice idea, but unfortunately will not work that way.
It was too beautiful to be true :)
I probably need to dig more into abstract factories, registration, etc. Two interesting questions from Stack Overflow:
I've prepared a valuable bonus for you!
Learn all major features of recent C++ Standards on my Reference Cards!
Check it out here: