Last Update:
Enhancing Visual Studio with Visual Assist
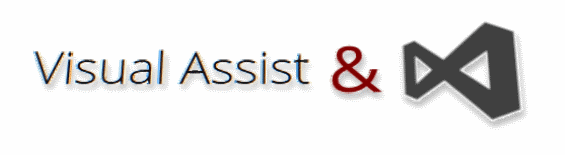
Table of Contents
How does your typical coding session in Visual Studio look like?
What’s the first thing you do when you’re about to start coding?
Yes… let’s check Gmail, Youtube, Reddit, etc… :)
OK, please be more professional!
So, let’s assume my Visual Studio (2013, 2015 or 2017) is already started. What to do next?
A quick session
Once I’ve finally checked all the Internet status of the World, I can
finally switch to Visual Studio and just look at the code. Today I am
about to write a new feature to my FileTest project. There’s one
interface called IFileTransformer
, and I’d like to add a new derived
class.
BTW: FileTest project is a real thing, take a look here - on my github page if you like.
Visual Studio opened not the file where the interface is located, so what to do? Let’s search the symbol.
Shift + Alt + S
and Visual Assist opens a nice window (Find
Symbol):
I can immediately go to the location of the interface now and recall what it does. This search symbol window is one of my essential tools; I can search almost everything with it. Of course, it works even if I remember just a part of the name.
There are some existing implementations of IFileTransformer
, so it’s
better to check what are they. I can, of course, skim the file
(fortunately all the code is in one header, but in a larger project
derived classes are usually spread over multiple files). With VA you can
just click on the type and use a powerful feature called Go To
Related, which shows all the derived classes for our interface.
Let’s now go to to the last derived class - MappedWinFileTransformer
.
We can simplify our life for now and just copy and paste it as a new
implementation. Let’s call it SuperFileTransformer
.
class SupaFileTransformer : public IFileTransformer
{
public:
using IFileTransformer::IFileTransformer;
virtual bool Process(TProcessFunc func) override;
};
Great… I have the class declaration in place, but I have to implement
the main method Process
. It’s another case where VA can help:
I can select the method and use VA to create an empty implementation. Most of the time it also places the code in the correct source file, at the end or near other methods of the same class.
But wait… haven’t I done a typo!? I wanted to write
SuperFileTransformer
, but I got SupaFileTransformer
. So let’s rename
it! In the previous screenshot you can see that there’s also an option
to Rename a symbol. So I use that, and immediately my new interface
has a correct name - and it’s changed across all the project!
You might notice that Visual Studio (even 2015) also contains that option, but VA offers it for much longer time, and it’s much reliable and faster as I’ve noticed.
Now, I don’t remember what’s the correct steps to implement the final file transformer, so for now, I just want to test if my simple code correctly works in the framework.
I can write a simple code like:
bool SuperFileTransformer::Process(TProcessFunc func)
{
const auto strInput = m_strFirstFile;
const auto strOutput = m_strSecondFile;
Logger::PrintTransformSummary(/*blocks */10, /*blockSize*/100,
strInput, strOutput);
return true;
}
It should only write a success log message.
Now I need to check and debug if everything works OK. F5 and then I’ll
try to set a breakpoint in my new code. One of the things that might
quite often interrupt the debugger session is stepping into an unwanted
function. There are ways to block that and for example, don’t enter
std::string
constructor… but with Visual Assist it’s super easy.
While debugging just use Step Filter. You can select which methods will be blocked.
For example, when I break at the code:
And I want to step into PrintTransformSummary
I’ll, unfortunately, go
into some strange code for std::string
. But then, VA will show
something like this on the Step Filter Window:
I can click on the marked debug event, and next time I won’t go into std::string constructor. This option can be saved per project or globally.
My code seems to work fine; the new interface was registered, it’s invoked correctly.
I’ll add a reminder to implement the read functionality:
// #todo: add final implementation
// #transformer: new, not finished implementation
Visual Assist has a great feature, added not that long ago, that transforms those hashtags into something searchable. Take a look:
In the Hashtags window, I can browse through my tags, see their location, read code around it. It’s great to leave notes, use it as bookmarks.
OK, I can now finish my little session and go back and check if the status of the World changed and open Reddit/Twitter/Youtube again!
A bit of intro
The story was a simple coding session. Let’s review all of the tools that helped me with code.
First of all the whole Visual Assist (VA):
It’s been several years since I started using Visual Assist. Every time I open Visual Studio without this powerful extension I just say “meh… “. Microsoft IDE is getting better and better, but still, there are areas where they cannot compete with VA.
Here’s a nice list of all the features compared to Visual Studio.
Compare Visual Assist to Microsoft Visual Studio
and here are the 10 Top Features
My main tool belt
Here are the tools that I am using all the time:
Find Symbol
Documentation: Find Symbol in Solution
It’s probably the most often feature I use. It allows me to find anything in the project.
I can search for all symbols, or just classes/structures. It offers filters, negative searches (with a hyphen), sorting. Not to mention it is super fast.
Go To Related/Go To Implementation
Documentation: GoTo Related, GoTo Implementation
It’s important when I want to switch between definition and declaration, see other implementations of some interface or a virtual method. What’s more, it also works from hashtags and comments.
Find All References
Documentation: Find References
Another core feature, when you want to search where a particular symbol is used. Visual Assist offers a better view of the results, take a look:
It’s grouped by file, with an option to show the code when you hover over a particular element.
VA Hashtags
Documentation: VA Hashtags
As I mentioned before, it allows you to have a little task tracker combined with bookmarks. Jump quickly between code sections, related modules, features. And everything is stored inside the same source file, no need to use external files, settings. It’s generated while editing.
Debug Step Filter
Documentation: VA Step Filter
Easily disable code that should be skipped while debugging. Simplifies debugging of code that touches some third party code, standard libraries, etc. Assuming that you don’t have to debug that far and can just focus on your code.
Refactoring/Code Generation:
Documentation: Refactoring, Code Generation
There are so many features here:
- rename
- change signature
- encapsulate field
- extract method
- introduce variable
- move implementation between files/headers
- move section to a new file
- create implementation
- create from usage
- create method Implementations
Syntax colouring
Documentation: Enhanced Syntax Coloring,
Just a taste of the syntax colouring.
Some little improvements
- Spellcheck - you can now write comments using proper words :)
- Snippets - I use it especially for comments for function/classes documentations, surround with a header guard, etc.
Other, there is even more cool stuff from Visual Assist, but I’m still improving my workflow and learning new things!
BTW: A few years ago I also wrote about hot VA can help in code understanding, take a look here: 3 Tools to Understand New Code from Visual Assist
You can see more on Whole Tomato Youtube Channel
Wrap up
Visual Assist improves my speed of coding, moving around the project files, understand what’s going on. I wanted to share my basic setup and core tools that I am using.
If you like Visual Assist or got a bit interested, just download the trial and play with it. I highly recommend this tool!
After the trial period it’s quite hard to live without it… believe me :)
I've prepared a valuable bonus for you!
Learn all major features of recent C++ Standards on my Reference Cards!
Check it out here: