Last Update:
A Gentle Intro to Developing C++ Apps for AWS and S3
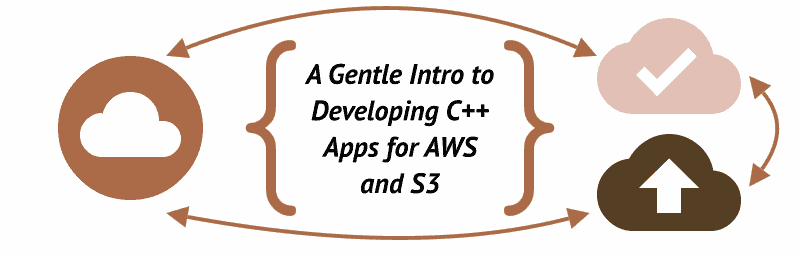
Table of Contents
Amazon Web Services (AWS) provide multiple tools for developing a native cloud application. In particular, the Software Development Kit (SDK) for C++ programming language enables developers to build powerful and efficient AWS applications for any platform. This includes Android, iOS, Linux and Windows apps.
This article introduces the basic concepts of C++ app development for AWS and S3, including real code examples.
This article is a guest post from Gilad David Maayan.
Gilad is a technology writer who has worked with over 150 technology companies including SAP, Samsung NEXT, NetApp and Imperva, producing technical and thought leadership content that elucidates technical solutions for developers and IT leadership. See his profile at Linkedin.
Why Developers Choose AWS?
Developers can use AWS for database storage, compute power, content delivery and other operations like:
-
Host dynamic websites on a server in the cloud
-
Store files in a secure environment
-
Store data in managed databases like PostgreSQL, Oracle or SQL Server
-
Deliver dynamic and static files using a CDN
-
Monitor your environments like CPU usage of RDS instances and trigger alarms
Reasons to Choose AWS C++ SDK for App Development
AWS launched the initial experimental version of an open-source SDK for C++ back in 2015. A developer preview version was released in March 2016. Finally, in September 2016, the SDK reached its production-ready 1.0 status.
The AWS SDK for C++ allows you to develop AWS applications using a modern C++ interface. It provides both low-level and high-level APIs for almost all AWS features. In addition, it decreases dependencies and provides platform portability on Linux, Windows, macOS, and mobile.
In their first announcement in 2015, Amazon highlighted that game developers are the main target of the C++ SDK. However, developers can also use it for systems engineering tasks and projects that require the efficiency of native code. This SDK makes it easier for developers and game studios to build code with hooks on AWS infrastructure.
In general, two main markets might benefit from the C++ SDK for AWS:
-
C++ game developers who want to build different 3D rendering engines, virtual reality apps, networking libraries and audio systems.
-
Development teams who require the power and efficiency of bare-metal programming languages like C++.
Getting Started with The AWS C++ SDK Development Environment
Developers can choose between Linux, Windows, Mac, iOS and Android as their operating systems. Integrated Development Environments (IDEs), like Visual Studio, are available for C++ development on AWS. In addition, the AWS SDK enables you to integrate Visual Studio with CMake, a tool for managing the software build process.
The list below specifies the necessary prerequisites to install the C++ SDK on your machine:
-
C++ compilers like Visual Studio starting from the 2015 version, GNU Compiler Collection (GNU) or Clang 3.3
-
At least 4 GB of RAM
-
For Linux, you need to have the header files for
libopenssl
,libcurl
,libuuid
,zlib
, andlibpulse
for Amazon Polly support
To install the C++ SDK on your machine, you can use the following methods:
Installation on Debian/Ubuntu systems
sudo apt-get install libcurl4-openssl-dev libssl-dev uuid-dev zlib1g-dev libpulse-dev
Installation on Redhat/Fedora systems
sudo dnf install libcurl-devel openssl-devel libuuid-devel pulseaudio-devel
Installation on CentOS systems
sudo yum install libcurl-devel openssl-devel libuuid-devel pulseaudio-libs-devel
Windows based-systems installation using NuGet
Microsoft Visual C++ developers can manage AWS C++ SDK dependencies using NuGet. To use this option, you have to install NuGet on your machine.
To use the SDK with NuGet
-
Open your Visual Studio project
-
Right-click your project name, and then select Manage NuGet Packages in the Solution Explorer
-
Search for a specific library name or service and select the packages you want. For instance, you can search for aws s3 native
-
Click on Install to install the libraries
Windows based-systems installation using Vcpkg
As an alternative to NuGet, you can use vcpkg to manage dependencies for AWS SDK for C++ projects that you develop with Microsoft Visual C++.
To use the SDK with vcpkg
-
Navigate to the vcpkg directory in the Windows command prompt
-
Integrate vcpkg into Visual Studio using the following command vcpkg integrate install
-
Install the AWS SDK C++ package with vcpkg install aws-sdk-cpp[*]:x86-windows --recurse
-
Open your Visual Studio project
-
Include the header files of AWS SDK for C++ in the source code
Providing AWS Credentials
To connect to any of the AWS services with the SDK, you must provide credentials. The AWS CLI and SDKs use provider chains to search for AWS credentials in different regions. This includes local AWS configuration files and system or user variables.
You can set your AWS credentials in multiple ways. The list below reviews the recommended approaches.
Using the AWS credentials profile file
The credentials file is located on your local machine at:
- ~/.aws/credentials on Mac, Linux or Unix
- C:\Users\USERNAME\.aws\credentials on Windows
The file contains the following format:
[default]
aws_access_key_id = personal_access_key_id
aws_secret_access_key = personal_secret_access_key
Change the personal\_access\_key\_id and personal\_secret\_access\_key
in the code to your own credentials.
Set the AWS\_SECRET\_ACCESS\_KEY
, the AWS\_ACCESS\_KEY\_ID
environment variables.
To set these variables on Linux, macOS, or Unix, use export :
export AWS\_ACCESS\_KEY\_ID=your\_access\_key\_id
export AWS\_SECRET\_ACCESS\_KEY=your\_secret\_access\_key
To set these variables on Windows.
set AWS\_ACCESS\_KEY\_ID=your\_access\_key\_id
set AWS\_SECRET\_ACCESS\_KEY=your\_secret\_access\_key
Using IAM roles
Specify an IAM role for the EC2 instances you plan to host your applications on. Make sure to include access to EBS Volumes if you’re using them. Once your role is created, you can give your instances and volumes access to that role. For more details, see IAM Roles for Amazon EC2 in the Amazon EC2 User Guide for Linux.
The AWS SDK for C++ loads the credentials automatically by using the default credential provider chain.
Tutorial: How to Create Amazon S3 Buckets with C++
After you’re done with the installation, you can develop C++ apps by leveraging different AWS services, such as S3. With S3, you can store files accessible by a range of services and applications. The following tutorial shows how to create Amazon S3 Buckets in any region. You can find the full code on this GitHub repository.
In S3, each bucket represents a folder of files or objects. Each bucket has its own unique name in the AWS ecosystem. The default option is to create buckets in the us-east-1 (N. Virginia) region.
Include the following files when developing S3 apps with the AWS SDK for C++
#include <aws/core/Aws.h>
#include <aws/s3/S3Client.h>
#include <aws/s3/model/CreateBucketRequest.h>
Define the main function variables
bool create_bucket(const Aws::String &bucket_name,
const Aws::S3::Model::BucketLocationConstraint ®ion = Aws::S3::Model::BucketLocationConstraint::us_east_1)
{
Set up the request for creating S3 buckets
The CreateBucket
method from the S3Client class is used to pass the CreateBucketRequest
with the name of the bucket.
Aws::S3::Model::CreateBucketRequest request;
request.SetBucket(bucket_name);
Check if the region is us-east-1
if (region != Aws::S3::Model::BucketLocationConstraint::us_east_1)
{
Specify the region as a location constraint
Aws::S3::Model::CreateBucketConfiguration bucket_config;
bucket_config.SetLocationConstraint(region);
request.SetCreateBucketConfiguration(bucket_config);
}
Create the S3 bucket
Aws::S3::S3Client s3_client;
auto outcome = s3_client.CreateBucket(request);
if (!outcome.IsSuccess())
{
auto err = outcome.GetError();
std::cout << "ERROR: CreateBucket: " <<
err.GetExceptionName() << ": " << err.GetMessage() << std::endl;
return false;
}
return true;
}
Additional Resources for C++ Developers on AWS
The following are online tutorials and resources for C++ developers on AWS.
- Introduction to the C++ Lambda Runtime—C++ implementation of the AWS Lambda runtime
- Developing an Android application with AWS SDK—building and application with the AWS SDK for C++, and running the app on an Android device.
Conclusion
The C++ language first appeared in the late 1970s. Some experts argue that C++ will fade out of existence due to the growing popularity of new languages and technologies. However, there is a growing demand in the industry for C++ development of virtual reality and 3D applications. AWS’ C++ SDK was designed to help developers meet this demand.
Hopefully, this article has helped you understand the first steps you should take to develop C++ apps in AWS using the SDK. With a little practice, you should be able to get your apps running in no time.
I've prepared a valuable bonus for you!
Learn all major features of recent C++ Standards on my Reference Cards!
Check it out here: