Last Update:
Beautiful C++: 30 Core Guidelines... Book Review
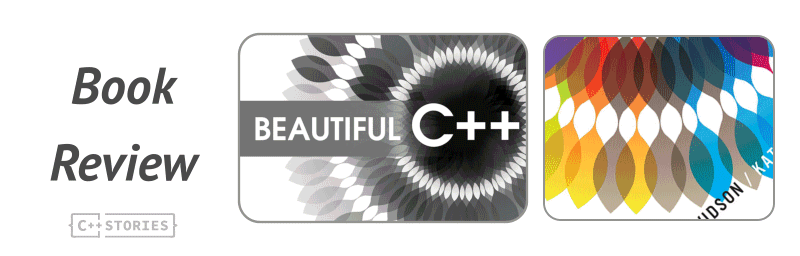
Today, I’ll show you my review of a cool book, “Beautiful C++,” written by two well-known C++ experts and educators: Kate Gregory and Guy Davidson. The book’s unique style gives us a valuable perspective on effective and safe C++ code.
Let’s see what’s inside.
Disclaimer: I got a free copy from the publisher.
Disclaimer 2: The links to Amazon are affiliate links, which provide me with some small commission.
The book
Here are the main links and info about the book:
The book on Amazon:
Beautiful C++: 30 Core Guidelines for Writing Clean, Safe, and Fast Code @Amazon (released December 16, 2021).
The book page at Pearson:
Beautiful C++: 30 Core Guidelines for Writing Clean, Safe, and Fast Code
All sample code is indexed at https://godbolt.org/z/cg30-ch0.0
For example, https://godbolt.org/z/cg30-ch1.1, for chapter 1, example 1.
Authors
J. Guy Davidson is a head of engineering practice at Creative Assembly, works on its Total War game franchise, curates its catalogue, and improves programming standards across its engineering team. He serves on the ISO C++ committee, moderates the #include <C++> discord server, speaks at C++ events, and offers C++ mentoring support through Prospela and BAME in Games.
Kate Gregory has 40+ years of development experience in multiple languages. She has keynoted on five continents, and volunteers in many C++ activities, especially #include <C++>, which is making the industry more welcoming and inclusive. Since 1986 she and her husband have run Gregory Consulting, helping clients worldwide become more effective.
Let’s see what’s inside the book.
The Structure
The main aim of the book is to explain the following selected tips from the C++ Core Guidelines:
The first part:
- P.2: Write in ISO Standard C++
- F.51: Where there is a choice, prefer default arguments over overloading
- C.45: Don’t define a default constructor that only initializes data members; use in-class member initializers instead
- C.131: Avoid trivial getters and setters
- ES.10: Declare one name (only) per declaration
- NR.2: Don’t insist to have only a single return-statement in a function
The second part:
- P.11: Encapsulate messy constructs, rather than spreading through the code
- I.23: Keep the number of function arguments low
- I.26: If you want a cross-compiler ABI, use a C-style subset
- C.47: Define and initialize member variables in the order of member declaration
- CP.3: Minimize explicit sharing of writable data
- T.120: Use template metaprogramming only when you really need to
Third:
- I.11: Never transfer ownership by a raw pointer (T*) or reference (T&)
- I.3: Avoid singletons
- C.90: Rely on constructors and assignment operators, not
memset
andmemcpy
- ES.50: Don’t cast away
const
- E.28: Avoid error handling based on global state (e.g. errno)
- SF.7: Don’t write using namespace at global scope in a header file
Fourth:
- F.21: To return multiple “out” values, prefer returning a struct or tuple
- Enum.3: Prefer class enums over “plain” enums
- ES.5: Keep scopes small
- Con.5: Use constexpr for values that can be computed at compile time
- T.1: Use templates to raise the level of abstraction of code
- T.10: Specify concepts for all template arguments
And the last one:
- P.4: Ideally, a program should be statically type safe
- P.10: Prefer immutable data to mutable data
- I.30: Encapsulate rule violations
- ES.22: Don’t declare a variable until you have a value to initialize it with
- Per.7: Design to enable optimization
- E.6: Use RAII to prevent leaks
The Core Guideline site contains over 300 tips, but the authors have only focused on approximately 10% of them.
In the introduction of the book, we can read:
The set we have chosen are not necessarily the most important. However, they are certainly the set that will change your code for the better immediately.
The book is split into five parts, and each part has six guidelines to help us understand the material more easily.
- Part one: Bikeshedding is bad - how to reduce the number of lines of your code and simplify it. For example, by using use in-class member initializers or avoiding getters and setters.
- Part two: Don’t hurt yourself - how to avoid messy code and minimize complexity. For example, by limiting explicit sharing of variables, proper use of ABI and more.
- Part three: Stop using that - some important bugs and bad C++ code style: singletons, casting away const or unsafe managing the ownership of resources.
- Part four: Use this new thing properly - efficient use of modern C++ style: enum classes, constexpr, templates.
- Part five: Write code well by default - good patterns for your code: type safety, immutable data structures avoiding uninitialized variables, and RAII.
In total, it’s around 300 pages + afterword and index.
If we look at a corresponding guideline like I.3: Avoid singletons you’ll see a short paragraph of introduction, some small example and a short discussion to alternatives. On the other hand, in the book, this guideline consists of 9 pages with unique examples, backstories, alternatives and discussions. The section shows things like:
- Why global objects are bad
- Singleton Design pattern overview
- Static initialization order fiasco
- Hiding singletons and making a namespace rather than a class
- And even how to use constexpr in some cases (
constexpr
is also covered in other sections)
To summarize, every core guideline has been significantly expanded from the original website text to enhance the experience of reading and learning.
My View
“Beautiful C++” is a fun and valuable read. You will not only learn good C++ style, but also hear lots of interesting and practical background stories. The book’s approach to teaching C++ is nothing short of innovative and memorable.
Throughout the book, you’ll find lots of interesting stories related to programming. One amusing anecdote is about a class that started off easy but gradually became more challenging, making the initialization process quite unstable. Another story revolves around the authors’ early experiences with computers and coding, such as using the ZX Spectrum, Atari, or powerful Motorola CPUs with 512MB of RAM. Additionally, there are some noteworthy tales behind the development of Rome: Total War, a game that was created by one of Guy’s employers.
By creating engaging stories around the concepts, authors manage to anchor the technical details into your memory. The bugs, notes, and intricate details become characters and events in a narrative, making them easier to recall and relate to.
Rather than attempting to be an exhaustive compendium of C++ knowledge, “Beautiful C++” wisely narrows its focus to 30 essential elements of the Core C++ Guidelines (There are 300+ topics out there…). This concentrated approach allows for a deep and insightful exploration of each element. The author’s commitment to explaining not just the ‘what’ but also the ‘why’ and ‘how’ of these elements offers readers a profound understanding.
You’ll probably ask and try to compare it with other books like Scott Meyers Effective C++ series. And it’s quite hard to compare them. Scott’s books (and others like Alexandrescu’s and Herb Sutters’s) were truely unique and innovative at that time. Back in late 90s or early 2000s, those books set the new standard and taught generations of C++ devs.
While Scott Meyers’ series and other seminal works have been the go-to resources for C++ best practices, they haven’t seen updates in a while, especially since Scott Meyers retired. This leaves a gap in the C++ literature, particularly when it comes to modern C++ standards like C++17 and C++20. “Beautiful C++: 30 Core Guidelines for Writing Clean, Safe, and Fast Code” steps in to fill this void. While the book won’t sound as revolutionary as those early 2000s guides, you’ll be content with the updates for recent C++ versions, new code style and lots of fresh C++ features.
An improvement that could enhance the value of this book (though it may increase the cost) would be the addition of color diagrams and syntax highlighting for the source code examples.
Summary
Final mark: 4.5+/5
Pros:
- Fun and easy to read!
- Can be read in any order,
- Use of storytelling,
- Concentrating on 30 key elements of C++ Core guidelines, so just good enough,
- Lots of backstories from gamedev and low-level coding,
- Easy to use examples at Compiler Explorer.
Cons:
- While some guidelines are covered thoroughly, you may notice that you have come across similar information in other books. As a result, they may not seem particularly innovative.
- Minor thing: I’d like to see full color version, not just black and white. That way the book would be really beautiful.
“Beautiful C++” offers a refreshing and unique take on C++ programming. Its ability to intertwine storytelling with technical instruction makes it stand out in from the crowd. This book is a must-read for anyone who wants to deepen their understanding of C++, value the aesthetics of coding, and transform their programming approach. I advise it for developers at all levels.
Get the book here: Beautiful C++: 30 Core Guidelines for Writing Clean, Safe, and Fast Code @Amazon.
And some other books to consider:
- More Exceptional C++: 40 New Engineering Puzzles, Programming Problems, and Solutions by Herb Sutter - 2001
- Amazon.com: C++ Coding Standards: 101 Rules, Guidelines, and Best Practices Alexandrescu & Sutter, 2004
- Effective Modern C++: 42 Specific Ways to Improve Your Use of C++11 and C++14, Scott Meyers - 2014
- C++ Core Guidelines Explained: Best Practices for Modern C++ by Rainer Grimm - 2022
Back to you
Have you read this book? What other books do you suggest to stay up to date with C++ Core guidelines?
Share your comments below.
I've prepared a valuable bonus if you're interested in Modern C++!
Learn all major features of recent C++ Standards!
Check it out here: