Last Update:
C++ Templates - The Complete Guide 2nd Book Review
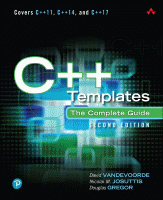
Table of Contents
A few months ago I received a quite massive mail package with something that was looking like a brand new C++ book :)
My initial plan was to review it quickly, maybe in one month. But I failed, as learning C++ templates is not that easy :) I needed much more time.
Time passed by and now I am ready for the review, so here you have it :) See my thoughts about the fantastic book on C++ templates, “the templates book” as many people call it.
Note: I got this book from the authors, but the review is not sponsored in any other form.
The Book
C++ Templates: The Complete Guide (2nd
Edition)
by David Vandevoorde, Nicolai M. Josuttis and Douglas Gregor
The main book’s site: www.tmplbook.com.
I own the printed copy, and it looks impressive:
The Structure
The book consists of 822 pages packed into 33 chapters!
There are three main parts:
- Basics
- Technical Details
- Templates and Design
Here’s the summary of the contents:
- Basics
- Function Templates
- Class Templates
- Nontype Template Parameters
- Variadic Templates
- Keyword
typename
, Zero Initialization, Templates for Raw Array and String Literals - Variable Templates and Template Template Parameters
- Move Semantics and
enable_if<>
- Template Template Parameters
- By Value or By Reference?
- Compile-Time Programming
- Using Templates in Practice
- Template Terminology
- Generic Libraries
This section should be probably read by every C++ programmer, as it discusses the underlying principles for templates: how they work and when we can use them. We go from simple function templates like
template <typename T>
T max(T a, T b) { ... }
And once the authors introduced background vocabulary and theory, we can move to class templates like:
template <typename T>
class Stack { ... };
The whole part adds more and more advanced topics, and it’s written in a tutorial style.
- Technical Details
- Declarations, Arguments, and Parameters
- Names and Parsing
- Instantiation
- Argument Deduction
- Specialization and Overloading
- Future Directions
In the second part, we dive into very advanced topics, and the book
becomes more a reference style. You can read it all, or focus on the
sections that you need.
In the “Future Directions” chapter there are topics related to upcoming
C++ highlights like modules, concepts.
- Templates and Design
- Static Polymorphism
- Traits and Policy Classes
- Type Overloading
- Templates and Inheritance
std::function<>
- Metaprogramming
- Typelists, Tuples, and Discriminated Unions
- Expression Templates
- Debugging Templates
After you have the basics and then you can jump into programming
techniques related to templates. The “traits” chapters are especially
handy as by learning how they are implemented you can efficiently learn
templates.
There’s also the “Debugging” chapter so you can learn techniques to make
your life easier when the compiler reports several pages of compiler
errors with templates :)
My View
This is a massive book!
I need to be honest with you: I still haven’t finished reading it (and it’s almost five months since I started). Such delay is, however, a very positive feature of the book because it’s not a “read-it-over-the-weekend” book. It’s filled with solid material and, let’s be clear, usually complicated stuff.
Probably the essential feature of this books is the relevance and that it is based on modern C++ - thus we have techniques from C++11, C++14 and of course C++17. What’s more, there are even topics about upcoming features, so you’ll be prepared for the future. The authors are ISO members with a vast amount of experience in C++, so you can be sure you get a very comprehensive material.
The first part - the basics - is written, as mentioned, in a tutorial
style, so you can just read it from the first chapter to the last and
gradually learn more and more. It starts with the basic samples and ends
with complex cases. A more advanced code sample is for example how to
implement call
that invokes a callable object and forward all the
input arguments to this object. Of course with variadic templates and
auto return type.
Then we have the third section - with so many real programming examples of how we can use templates.
For example one month ago I was on a local C++ User Group Krakow meeting (link here) and there was a great live coding by Tomasz Kaminski about implementing tuples. I think that if you know how to implement tuples, then you’re quite a template expert :) Here, in the book, you have a separate chapter on the topic of tuples. I could read it and slowly try to understand what’s going on.
Summary
Final mark: 5/5 + Epic Badge! :)
An epic book that will fill a lot of your time and will leave you with solid knowledge about modern C++ templates (including C++11, C++14 and C++17… and even some insight about upcoming things in C++20). What can I say more? :)
What’s more, I can add that the link to the book was posted at r/cpp and it wasn’t downvoted. In one comment someone said that this book (also the first version) is considered as “the template book”
See the full thread at r/cpp/tmplbook2
You can also see a good presentation by N. Josuttis (one of the authors) that happened in the recent ACCU 2018, where Nicolai talks about how the book was written (and a bit about the first edition):
To sum thing up: if you want to learn templates, here is the book for you :)
Let me know what do you think about it.
- Have you seen it already?
- What other resources do you use to learn about C++ templates?
I've prepared a valuable bonus if you're interested in Modern C++!
Learn all major features of recent C++ Standards!
Check it out here: