Last Update:
How to Use C++ for Azure Storage
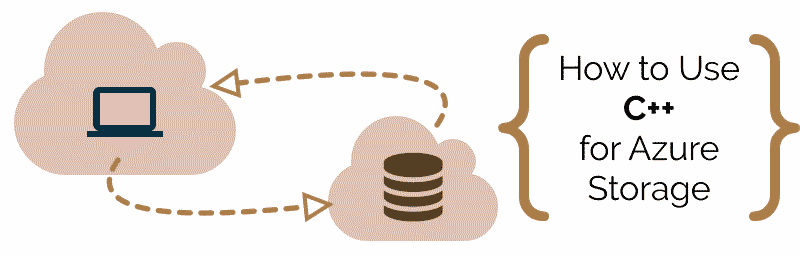
Table of Contents
Blob storage is an object storage service you use in Azure. It is designed for storing large volumes of unstructured data, including text, binary data, images, and text. In this service, your data is stored in containerized blobs with a directory-like structure. You can use blob storage to ensure flexible access to storage, high availability, and data consistency. Read on to learn how you can use C++ with Azure storage.
This article is a guest post from Gilad David Maayan.
Gilad is a technology writer who has worked with over 150 technology companies including SAP, Samsung NEXT, NetApp and Imperva, producing technical and thought leadership content that elucidates technical solutions for developers and IT leadership. See his profile at Linkedin.
David also wrote another article for this site: A Gentle Intro to Developing C++ Apps for AWS and S3
Azure Blob Storage Use Cases and Tiers
Use cases for blob storage include:
- Audio and video streaming
- Content delivery
- Logging and log aggregation
- Data analysis and big data storage
- Archive and backup storage
- Disaster recovery
When using blob storage, there are three storage tiers you can choose from:
- Hot Access Tier—intended for frequently accessed data or data requiring low-latency. It exchanges higher storage costs for lower access costs.
- Cool Access Tier—intended for infrequently accessed data that you need to store for 30 days or more. It exchanges lower storage costs for higher-latency and access costs.
- Archive Access Tier—intended for data that is infrequently or never accessed that you need to store for 180 days or more. It exchanges low storage costs for limited, higher-cost data access.
Azure Storage Client Library for C++
When you need to access or manage blob storage and are working in C++, you can use the Azure Storage Client Library for C++. This library enables you to more easily build and integrate applications written in C++ with blob storage services.
Features of the library include:
- Tables—includes capabilities for creating and deleting tables and for creating, querying, reading, updating, and deleting entities.
- Blobs—includes capabilities for creating, reading, updating, and deleting blobs.
- Queues—includes capabilities for creating and deleting queues, inserting and peeking at queue messages, and advanced queue operations.
- Files—includes capabilities for creating, deleting and resizing shares, creating and deleting directories, and creating, reading, updating, and deleting files.
Configuring Your C++ Application to Access Blob Storage
When building your applications, you can use the following steps to attach your application to your blob storage resources. If you haven’t migrated to Azure yet, you can find more information in this guide about Azure migration.
1. Include libraries
To add libraries, you need to include the following statements in your code file:
#include <was/storage_account.h>
#include <was/blob.h>
#include <cpprest/filestream.h>
#include <cpprest/containerstream.h>
You can find more information about this code in the official documentation.
2. Setup a Connection String
Next, you need to set up a storage connection string. A connection string enables you to store credentials and endpoint information needed to access data management services. When configuring your connection string, you need to include the name of your storage account and your access keys. These are listed in your Azure Portal.
You can model your connection string on the following example:
const utility::string_t storage_connection_string(U("DefaultEndpointsProtocol=https;AccountName={Account Name};AccountKey={Account Access Key}"));
3. Retrieve Your Storage Account
Once your connection string is created, use the following parse method to retrieve your storage account information. Within this method, cloud_storage_account represents your specific account information.
azure::storage::cloud_storage_account storage_account =
azure::storage::cloud_storage_account::parse(storage_connection_string);
After your information is retrieved, you can retrieve your individual objects by creating a cloud_blob_client class. You can do this with the following lines:
azure::storage::cloud_blob_client blob_client = storage_account.create_cloud_blob_client();
Performing Common Operations on Blob Storage Using C++
Once your application is connected to your blob storage, you can begin performing a variety of common operations. Some of the most useful operations are covered below.
Uploading Data
Within Blob storage, you can store page and block blobs; block is the recommended type. To upload data to block blobs, you need to obtain your blob reference via a container reference. Once you have a blob reference, you can upload data via the upload_from_stream method. You can use this method to create new blobs or to modify existing blobs.
To see how this works, refer to the following code. Keep in mind, this assumes that you already have an existing container, have retrieved your storage account, and created a blob client (as s covered above).
To reference your container:
azure::storage::cloud_blob_container container =
blob_client.get_container_reference(U({Container Name}));
To retrieve a reference to your blob:
azure::storage::cloud_block_blob blockBlob =
container.get_block_blob_reference(U({Blob Name}));
To create or overwrite your blob:
concurrency::streams::istream input_stream = concurrency::streams::file_stream<uint8_t>::open_istream(U({Text File Name})).get();
blockBlob.upload_from_stream(input_stream);
input_stream.close().wait();
Downloading Blobs
To retrieve data stored in blobs, you need to convert your blob data to a stream object which you can then transfer to a local file. You can accomplish this with the download_to_stream
method.
To perform this action, you need to first retrieve your storage account, create a blob client, and retrieve your container and blob references (as shown above). Once these processes are complete, you can use the following to download your blob data:
concurrency::streams::container_buffer<std::vector<uint8_t>> buffer;
concurrency::streams::ostream output_stream(buffer);
blockBlob.download_to_stream(output_stream);
std::ofstream outfile({Text File Name}, std::ofstream::binary);
std::vector<unsigned char>& data = buffer.collection();
outfile.write((char *)&data[0], buffer.size());
outfile.close();
Deleting Blobs
To delete your blobs, you need to determine your blob reference. Once you know the reference, you can use blockBlob.delete_blob();
to delete it. Keep in mind, that this process permanently deletes your blobs so you should use it with care.
Conclusion
You can easily use C++ for Azure storage operations, with the Azure Storage Client Library for C++. Once you include Azure’s premade libraries in your code, you can set up a connection string that enables storage of endpoint and credentials data. You should then retrieve your storage account with the parse method provided above. Once C++ is properly configured, you’ll be able to upload data, download blobs, delete blobs, and perform many other actions.
I've prepared a valuable bonus for you!
Learn all major features of recent C++ Standards on my Reference Cards!
Check it out here: