Last Update:
Five Awesome C++ Papers for the Q2 2021 and C++23 Status
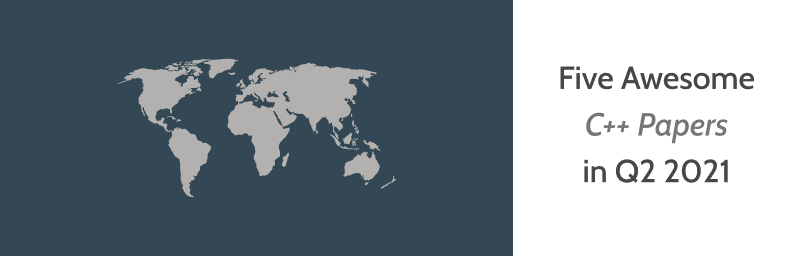
The work on C++23 continues! Without the face-to-face meetings, the Committee gathers online and discusses proposals and new additions to the language. See my latest report on what changed in C++ in April, May, and June 2021.
Let’s start!
Disclaimer: the view presented here is mine and does not represent the opinion of the ISO C++ Committee.
The online meetings
Until March 2020, the ISO Committee met “offline” two or three times per year. The gatherings took place in various locations, mainly in Europe and the USA. Have a look at my few previous reports:
- Five Awesome C++ Papers for the Prague ISO Meeting and C++20 Status
- Five Awesome C++ Papers for the Belfast ISO Meeting and C++20 Status
- Five Awesome C++ Papers for Cologne ISO Meeting
Since March 2020, due to pandemics, the meeting went entirely online. In the last year, around 200 virtual calls were organized! As you can see, the Committee is alive and working already on C++23 and C++20 fixes :)
Have a look at the most recent summary from Herb Sutter:
and some old ones, also from Herb Sutter:
- Trip report: Autumn ISO C++ standards meeting (virtual)
- Trip report: Winter 2021 ISO C++ standards meeting (virtual)
If you want to know more about the online process, have a look at this document which describes how the ISO Committee works right now:
P2145R1: Evolving C++ Remotely
Upcoming Meetings
Due to the global pandemic situation, ISO postponed all the face-to-face meetings, but hopefully, they will return in 2022. The next place for the conference is in Portland, USA, and later New York.
Here’s the plan for the meetings at the ISO C++ website: Upcoming Meetings, Past Meetings: Standard C++
The status of C++23
The C++20 Standard is already published (Dec 2020), and since mid-2020, C++ Experts started the work on C++23. The papers and votings are now asynchronous. Thus far, the following features were accepted:
Recently added into C++23
- P1938 -
if consteval
feature to C++23 - this fixes the interaction betweenconsteval
andstd::is_constant_evaluated()
(a magic function). - P1401 - Narrowing contextual conversions to bool - narrowing is not allowed in contextual conversion to
bool
in core constant expressions, this paper lifts this requirement forif constexpr
andstatic_assert
and should make code more readable. - P1132 -
out_ptr
andinout_ptr
abstractions to help with potential pointer ownership transfer when passing a smart pointer to a function that is declared with aT**
“out” - P1659 generalizes the C++20
starts_with
andends_with
onstring
andstring_view
by adding the general formsranges::starts_with
andranges::ends_with
. - P2166 - Prohibit
std::basic_string
andstd::basic_string_view
construction fromnullptr
.
Features already available:
- Literal suffix for (signed)
size_t
- we can now writeauto i = 0zu
which createssize_t
variable. - Make
()
more optional for lambdas - no need to use()
with lambdas with capture and mutable:[&var] mutable {}
. This also applies to trailing return types and other specifiers. - The stack trace library - additional debugging information, similar to other languages like Python, C#, or Java.
<stdatomic.h>
std::is_scoped_enum
contains()
forbasic_string
/basic_string_view
std::to_underlying
- yep, this is almost the same utility that Scott Meyers proposed in his Effective Modern C++ Book :) Item 10, on scoped enums.std::visit
for classes derived fromstd::variant
- Relaxing requirements for
time_point<>::clock
As always, you can see their status on this great cppreference page:
And here’s the overall plan for C++23:
To boldly suggest an overall plan for C++23
Awesome papers
Let’s now have a look at some recent papers listed for the Second Quarter of 2021.
Here are the links for:
The first one:
Making std::unique_ptr constexpr
Some time ago, I wrote a blog post on constexpr
dynamic memory allocations - see at: constexpr Dynamic Memory Allocation, C++20 - C++ Stories.
Unfortunately, in C++20, it requires raw memory management as you cannot use smart pointers and rely on RAII.
For example this code:
constexpr auto fun() {
auto p = std::make_unique <int>(4);
return *p;
}
constexpr auto i = fun();
static_assert(4 == i);
It won’t compile.
The proposal aims to fix this issue so that we could use smart pointers - only unique_ptr
to be precise - in the constexpr
context. The author also discusses some challenges when trying to implement shared_ptr
this way.
Support for UTF-8 as a portable source file encoding
In short:
We propose that UTF-8 source files should be supported by all C++ compilers.
This change would allow having more portable compilation across many compilers and platforms. Currently, each compiler handles encoding independently, and sometimes it might lead to unwanted consequences and encoding issues.
Stacktrace from exception
Stacktrace is an utility added and approved for C++23, the proposal extends the library so that you can use: std::stacktrace::from_current_exception()
and tat way get more information that exception.what()
.
Here’s the example code from the paper:
try {
foo("test1");
bar("test2");
} catch (const std::exception& exc) {
std::stacktrace trace = std::stacktrace::from_current_exception(); // <<
std::cerr << "Caught exception: " << exc.what() << ", trace:\n" << trace;
}
As you can see, with this code, you could quickly get what’s the current call stack and be able to debug the code much more straightforward.
std::expected
10th (12th) iteration of this paper!
To be precise (as I got a comment @reddit, from sphere991 )
P0323R10 isn’t the 10th iteration, it’s the 12th. The first was N4109 and then R0 thru R10 is the next 11. Yes, this proposal recently turned 7 years old.
Currently, you can use many different strategies to inform about an error from a function:
- exceptions
- output parameters and return codes
std::optional
- see Error Handling and std::optional - C++ Stories- and more
with std::expected
you could write:
std::expected<Object, error_code> PrepareData(inputs...);
And that way, convey more data to the caller in case of an error.
The expected object works similarly to std::variant<ReturnType, Error>
- so only one part will be available to the caller. If there’s no error, then you can just fetch the value.
Mark all library static cast wrappers as [[nodiscard]]
It’s a short paper, just 2 pages, but stresses the importance of [[nodiscard]]
and adding it to even more places in the Standard Library.
For example if you perform a “language” based cast and forget to use the value, then you’ll get a compiler warning:
val;
static_cast<T &&>(val); // gives warning
std::move(val); // no warning!
The paper suggest adding [[nodiscard]]
to the following functions:
to_integer
forward
move
- yep,move()
doesn’t move :) it’s a cast to r-value reference.move_if_noexcept
as_const
to_underlying
identity
bit_cast
Your Turn
What are your favorite proposals that might be included in the next C++ Standard?
Share your thoughts in the comments below the article.
I've prepared a valuable bonus for you!
Learn all major features of recent C++ Standards on my Reference Cards!
Check it out here: